SDK Integration Steps
Platform Support
CRUX supports the following platforms:
- Web - https://github.com/cruxprotocol/js-sdk
- Electron apps - https://github.com/cruxprotocol/js-sdk
- React Native - https://github.com/cruxprotocol/rn-sdk
- Java - https://github.com/cruxprotocol/android-sdk
Upcoming:
- Swift (Native iOS)
This page is intended to help CRUX Clients estimate the development effort involved in integrating CRUX. This page is divided into 2 sections
PHASE 1: CRUXPay - Human readable names for addresses
PHASE 2: CRUXGateway - Interoperability between wallets, services and dApps
PREREQUISITE
Please read https://docs.cruxpay.com/docs/quickstart for installation instructions.
PHASE 1: CRUXPay Protocol Integration
CRUXPay Protocol requires 2 UX flows to be developed
Prerequisites
The wallet needs to initialise CruxClient from the CRUX SDK with a new dedicated Private Key on behalf of the User. This Private Key is referred to as "CRUX ID Owner Key". This key is required to make any modifications or to prove identity as the owner of a particular CRUX ID.
The wallet needs to create, configure, and own a 'walletClientName'. Anyone can create a walletClientName. CRUX has implemented a helpful interface to do so at https://cruxpay.com/wallet/dashboard.
You can use cruxdev
as walletClientName if you don't have one at the moment.
import { CruxPay } from "@cruxpay/js-sdk";
let cruxClient = new CruxPay.CruxClient({
walletClientName: 'testwallet',
privateKey: '...'
});
import com.crux.sdk.CruxClient;
import com.crux.sdk.model.*;
CruxClientInitConfig.Builder configBuilder = new CruxClientInitConfig.Builder()
.setWalletClientName("testwallet")
.setPrivateKey("...");
CruxClient cruxClient = new CruxClient(configBuilder, androidContextObject);
Now that CruxClient is initialized and bound to a Private Key, it is ready to do all operations.
Flow 1 - Pay to CRUX ID Flow
The first and most basic operation is the when a User wants to pay to another User's CRUX ID.
The injected Private Key is not utilised for this operation.
This flow is best represented at the 'Payment' screen of the wallet, where Users typically enter a recipient cryptocurrency address
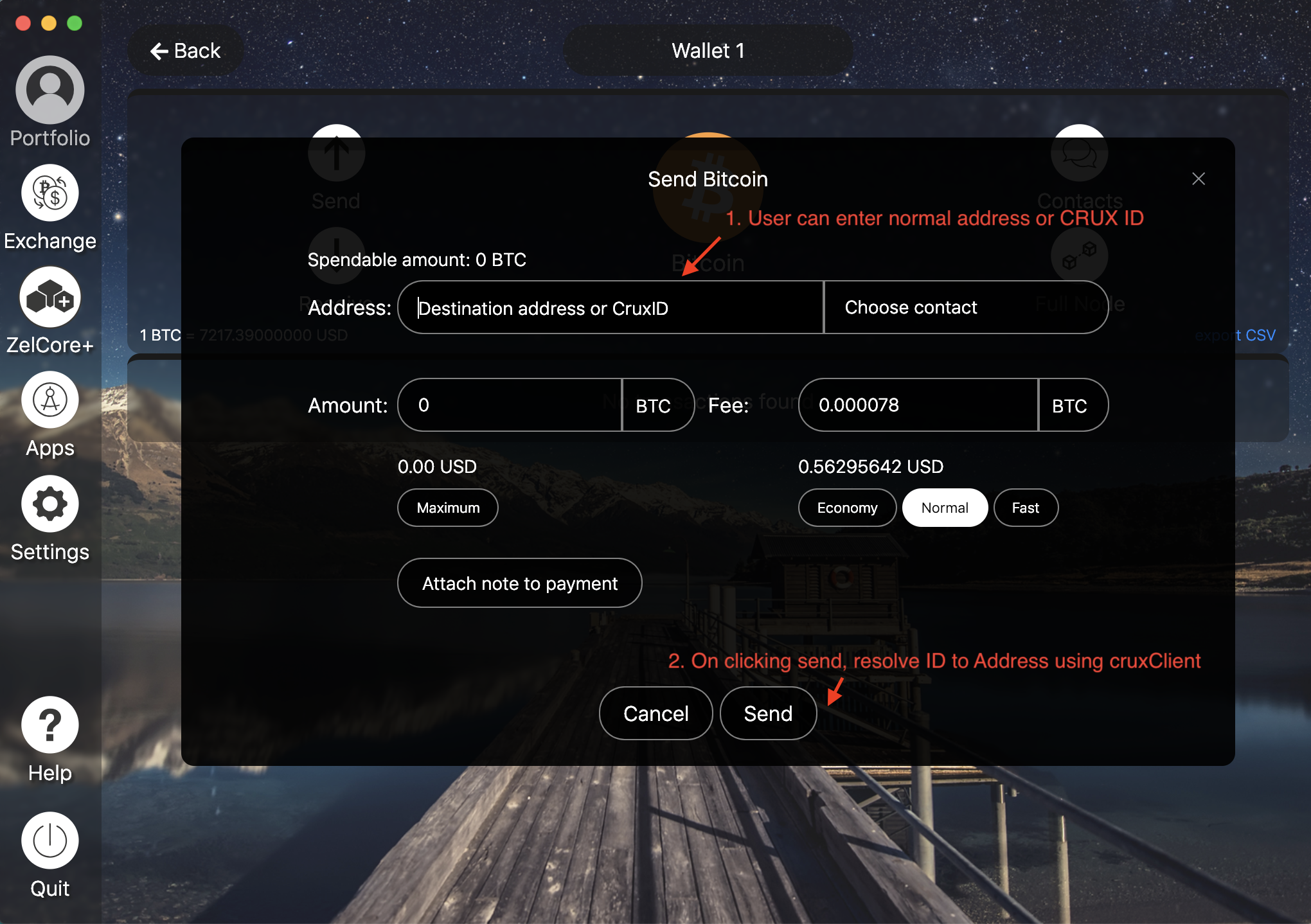
cruxClient.resolveCurrencyAddressForCruxID('[email protected]','btc').then((address) => {
console.log(address);
})
// NOTE: The symbol 'btc' is sent as the second parameter. How does CRUX know what 'btc' is? This is what the Wallets configure against 'walletClientName' at the CRUXPay Wallet dashboard.
cruxClient.resolveCurrencyAddressForCruxID(
"[email protected]", "xrp",
new CruxClientResponseHandler<CruxAddress>() {
@Override
public void onResponse(CruxAddress successResponse) {
System.out.println(successResponse);
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
System.out.println(failureResponse);
}
}
);
// NOTE: The symbol 'btc' is sent as the second parameter. How does CRUX know what 'btc' is? This is what the Wallets configure against 'walletClientName' at the CRUXPay Wallet dashboard.
Flow 2 - ID Management Flow
The second flow to work on is CRUX ID Management. This is the flow presented to the user in order to create a new CRUX ID and to modify their existing CRUX ID.
Here we display the current CRUX ID State. We also expose UI to create or edit CRUX IDs.
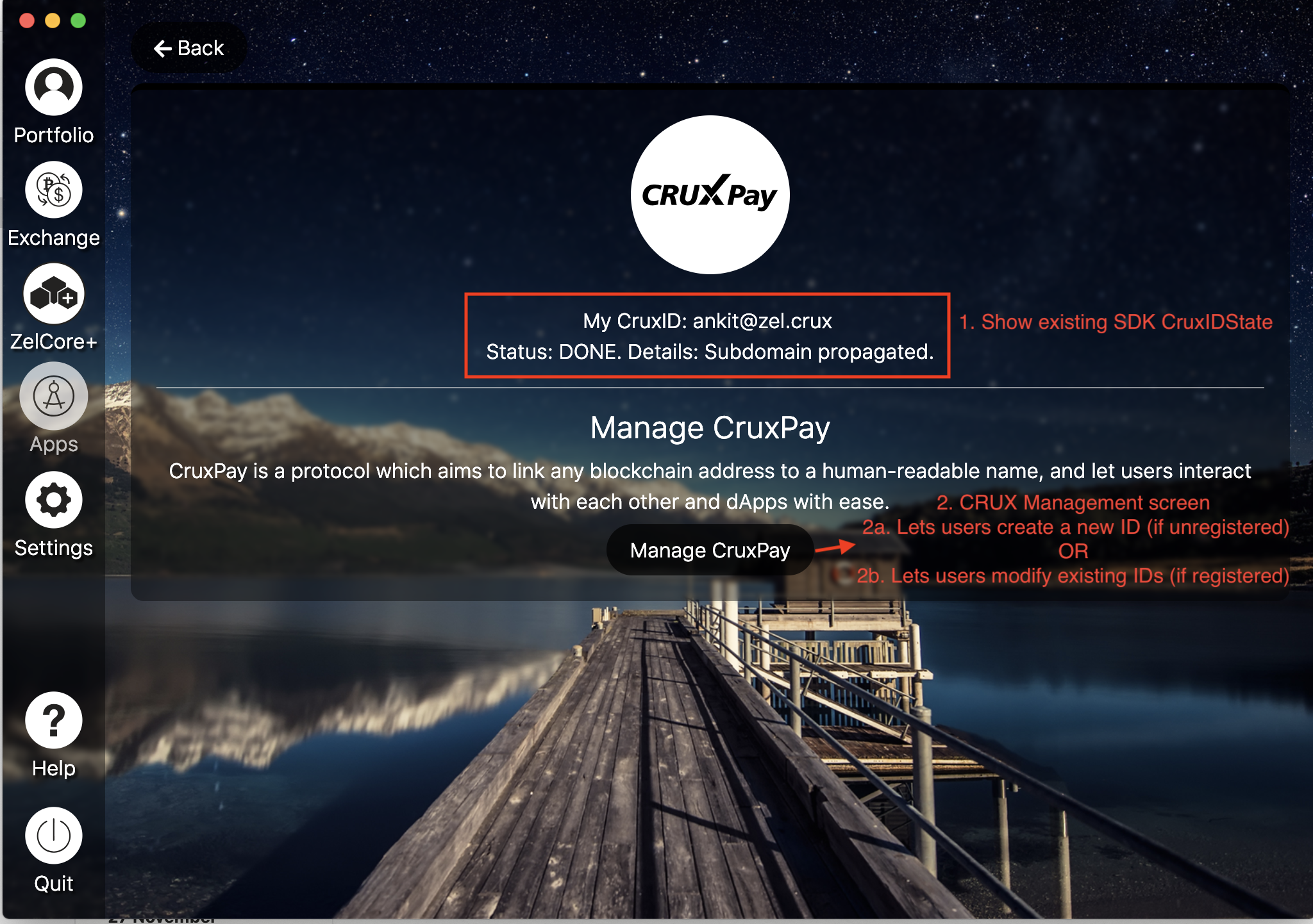
cruxClient.getCruxIDState().then((cruxIDState) => {
// cruxIDState.cruxID is null if unregistered. If registered, it will contain the ID
})
cruxClient.getCruxIDState(
new CruxClientResponseHandler<CruxIDState>() {
@Override
public void onResponse(CruxIDState successResponse) {
// cruxIDState.cruxID is null if unregistered. If registered, it will contain the ID
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
}
}
);
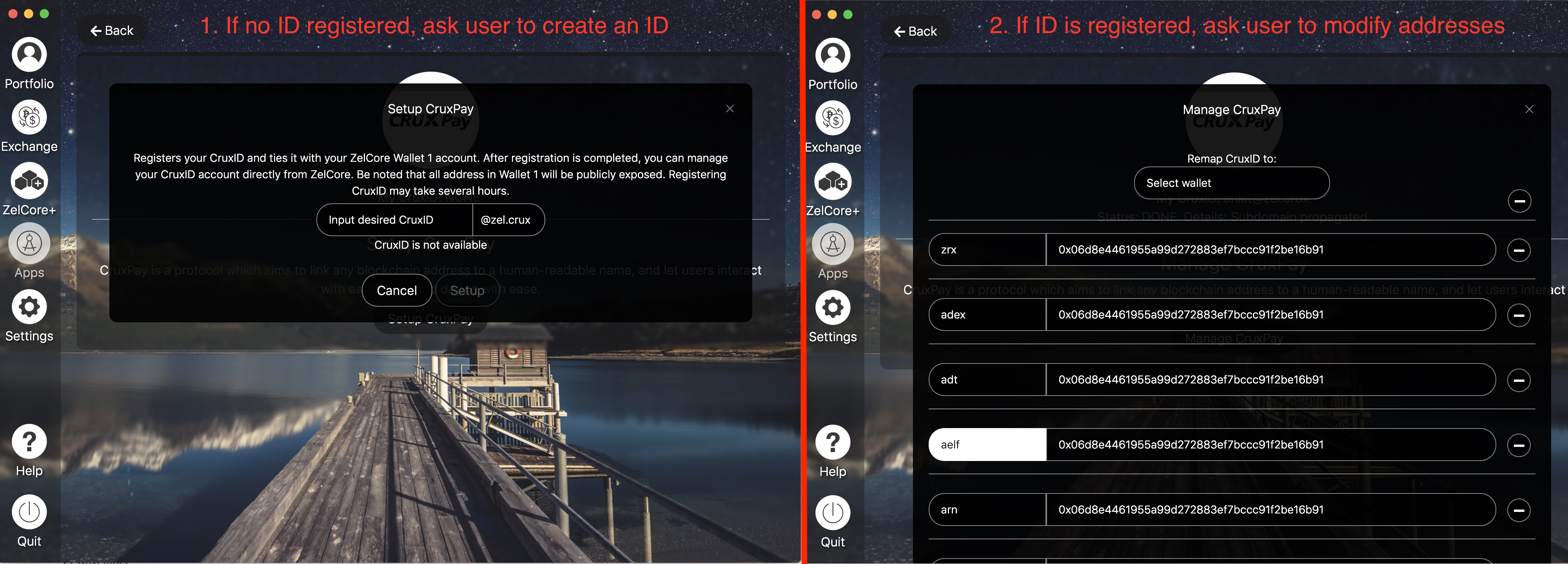
LEFT: User not registered
RIGHT: User already registered
// ------ LEFT: User not registered -----
// We want to allow users to choose and register a CRUX ID
cruxClient.isCruxIDAvailable('mynewid').then(){
// This will check if mynewid is available to be registered on above injected `walletClientName`
// we injected `testwallet` above. So - [email protected]
}
cruxClient.registerCruxID('mynewid').then((result) => {
// This will register [email protected]
})
// ------ RIGHT: User already registered -----
// We want to allow users to manage data associated to their ID
cruxClient.getAddressMap().then((result)=>{
// result will contain all public addresses of the user bound to their CRUX ID
})
// User can remove/add/modify any addresses and currencies in their wallet. It can be written securely to their CRUX ID with -
cruxClient.putAddressMap({
'btc': {
'addressHash':'1z2...42a'
},
'eth' {
'addressHash':'0x12z2...2d'
}
})
// ------ LEFT: User not registered -----
// We want to allow users to choose and register a CRUX ID
client.isCruxIDAvailable('mynewid',
new CruxClientResponseHandler<Boolean>() {
@Override
public void onResponse(Boolean successResponse) {
// This will check if mynewid is available to be registered on above injected `walletClientName`
// we injected `testwallet` above. So - [email protected]
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
}
}
);
cruxClient.registerCruxID("mynewid",
new CruxClientResponseHandler<Void>() {
@Override
public void onResponse(Void successResponse) {
// This will register [email protected]
// ID Will be owned by the injected private key
// New IDs take 6-8 confirmations in the Bitcoin network to confirm.
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
}
}
);
// ------ RIGHT: User already registered -----
// We want to allow users to manage data associated to their ID
// See user's existing public addresses
client.getAddressMap(
new CruxClientResponseHandler<HashMap<String, CruxAddress>>() {
@Override
public void onResponse(HashMap<String, CruxAddress> successResponse) {
// result will contain all public addresses of the user bound to their CRUX ID
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
}
}
);
private HashMap<String, CruxAddress> getCurrencyMap() {
HashMap<String, CruxAddress> currencyMap = new HashMap<String, CruxAddress>();
currencyMap.put("btc", new CruxAddress("1HX4KvtPdg9QUYwQE1kNqTAjmNaDG7w82V", null));
currencyMap.put("eth", new CruxAddress("0x0a2311594059b468c9897338b027c8782398b481", null));
currencyMap.put("tron", new CruxAddress("TG3iFaVvUs34SGpWq8RG9gnagDLTe1jdyz", null));
currencyMap.put("xrp", new CruxAddress("rpfKAA2Ezqoq5wWo3XENdLYdZ8YGziz48h", "7777"));
return currencyMap;
}
// User can remove/add/modify any addresses and currencies in their wallet. It can be written securely to their CRUX ID with -
client.putAddressMap(getCurrencyMap(),
new CruxClientResponseHandler<CruxPutAddressMapSuccess>() {
@Override
public void onResponse(CruxPutAddressMapSuccess successResponse) {
}
@Override
public void onErrorResponse(CruxClientError failureResponse) {
}
}
);
PHASE 2: CRUX Gateway Integration
[upcoming]
Updated over 5 years ago